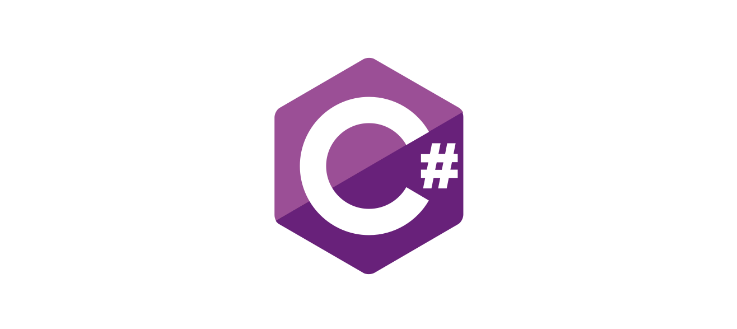
SignalR, developed by Microsoft, enables real-time communication in .NET-based web applications. In this article, we will explore the fundamental concepts of SignalR, its advantages, and demonstrate its usage through an application example.
Introduction
Real-time communication allows users to access updated data instantly in web applications. SignalR is a library designed to meet this need.
Fundamental Concepts
1. Hub: Central component of SignalR facilitating communication between the server and clients. A hub contains server-side logic and notifications to be sent to clients.
2. Client: Represents the user’s browser or application. With SignalR, clients can connect to the server and respond to real-time data by subscribing to specific events.
3. Connection: SignalR communicates through connection points. When a connection is established, data exchange between clients and the server begins.
Advantages
1. Real-Time Communication: Enables data to be updated instantly, allowing the creation of real-time applications.
2. Bidirectional Communication: Allows the server to send data to clients, and vice versa, facilitating the development of interactive applications.
3. Various Transport Mechanisms: Supports various transport mechanisms such as WebSockets, Server-Sent Events (SSE), and Long Polling, providing flexibility to work in different environments.
Application Example: Live Chat
Let’s examine the usage of SignalR through an example of a live chat application.
Server (Hub Creation)
using Microsoft.AspNetCore.SignalR;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
Client (JavaScript):
<!-- Application HTML and JavaScript section -->
<script>
const connection = new signalR.HubConnectionBuilder()
.withUrl("/chatHub")
.configureLogging(signalR.LogLevel.Information)
.build();
connection.start().then(function () {
console.log("Connected to SignalR Hub");
}).catch(function (err) {
return console.error(err.toString());
});
connection.on("ReceiveMessage", function (user, message) {
const encodedMessage = user + ": " + message;
const li = document.createElement("li");
li.textContent = encodedMessage;
document.getElementById("messagesList").appendChild(li);
});
function sendMessage() {
const user = document.getElementById("userInput").value;
const message = document.getElementById("messageInput").value;
connection.invoke("SendMessage", user, message).catch(function (err) {
return console.error(err.toString());
});
}
</script>
In this example, a live chat application is simulated. When a user enters their username and message, clicking the “Send” button instantly relays the message to other connected users.
Conclusion
SignalR is a powerful and flexible tool for developing real-time applications. In this article, we’ve provided an extensive overview of SignalR’s fundamental concepts and demonstrated its usage through an application example. Depending on your application scenarios, SignalR can be employed to create interactive and dynamic web applications.