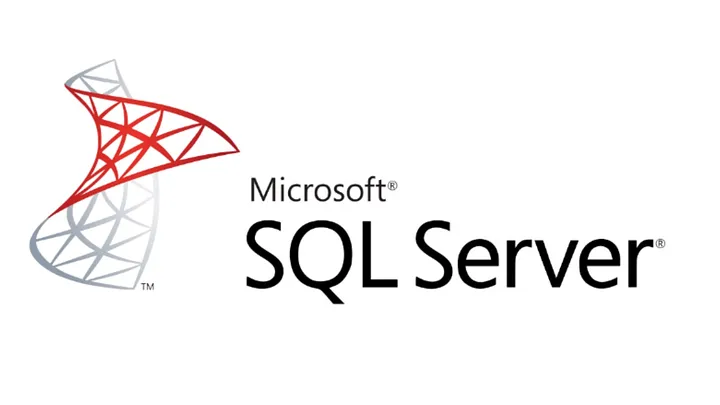
MSSQL offers a range of powerful features to perform complex database operations. One of these features is cursors. Cursors enable navigation and control over data within a database table. Cursors are ideal for scenarios where data needs to be processed row by row and sequential operations are required. In this article, we will discuss the basic principles of cursor usage in MSSQL and some best practices.
I. What is a Cursor?
In MSSQL, a cursor is a data structure that allows step-by-step traversal and control over a result set returned by a query. A cursor is used to process each row in the result set. A set of operations can be performed on each row, and the cursor advances row by row. By using a cursor within a loop, each subsequent row is processed and operations are performed.
II. Cursor Usage:
The usage of a cursor in MSSQL generally involves the following steps:
Cursor Creation: The first step is to create a cursor. During cursor creation, a query is specified, and the result set of that query is assigned to the cursor.
Cursor Opening: After the cursor is created, it is opened and used to traverse the result set. Additionally, filtering or sorting options can be specified as needed.
Fetch: Fetch allows the cursor to move to the next row and return the data of that row. The fetch operation is repeated until the end of the cursor or until a specific condition is met.
Performing Operations: After the fetch operation, the required operations are performed on each row. Operations can include database updates, calculations, or data processing tasks.
Cursor Closing and Cleanup: When cursor usage is completed, the cursor is closed and cleaned up. This step ensures the release of database resources and is important for performance.
Example:
-- Cursor creation
DECLARE @CustomerId INT
DECLARE @CustomerName VARCHAR(50)
DECLARE CustomerCursor CURSOR FOR
SELECT CustomerId, CustomerName FROM Customers
-- Cursor opening
OPEN CustomerCursor
-- Fetching the first row
FETCH NEXT FROM CustomerCursor INTO @CustomerId, @CustomerName
-- Performing operations row by row
WHILE @@FETCH_STATUS = 0
BEGIN
-- Perform operations here
PRINT 'CustomerID: ' + CONVERT(VARCHAR(10), @CustomerId)
PRINT 'CustomerName: ' + @CustomerName
PRINT '---------------------------'
-- Fetching the next row
FETCH NEXT FROM CustomerCursor INTO @CustomerId, @CustomerName
END
-- Closing and deallocating the cursor
CLOSE CustomerCursor
DEALLOCATE CustomerCursor
III. Best Practices: When using a cursor in MSSQL, it is important to follow these best practices:
- Before using a cursor, review alternative approaches such as query or set-based methods for performing database operations. Cursor usage should be avoided when unnecessary, as it can impact performance.
- When opening and closing a cursor, ensure that all necessary options are specified. Properly adjusting parameters such as filtering, sorting, and result set size is important.
- When performing fetch operations, retrieve larger sets of data whenever possible to minimize unnecessary database access. This helps reduce the cost of data retrieval.
- When performing operations, try to perform bulk operations such as database updates. Performing batch updates with a single query or operation is more efficient than individual update operations.
- Remember to close and clean up the cursor when cursor usage is completed. This ensures proper release of database resources and avoids performance issues.
Cursor usage in MSSQL provides a useful tool for gaining more control and performing complex operations in database transactions. However, it should be used with caution due to its potential impact on performance. In this article, we discussed the basic principles of cursor usage and best practices in MSSQL. When using cursors, it is important to configure cursor settings and operations according to the requirements of your database transactions.
I hope you find this article helpful. Happy coding!