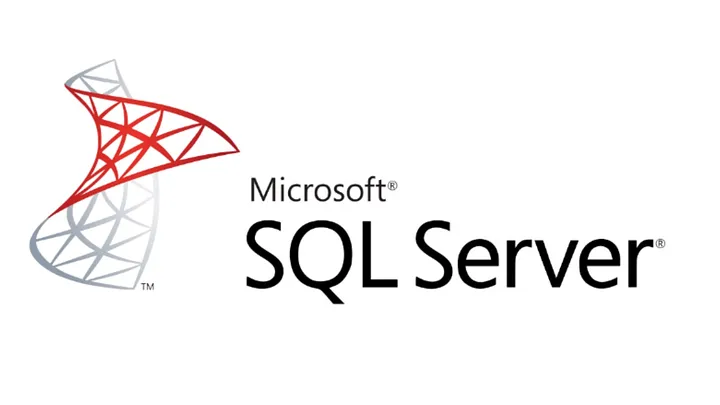
Using WITH
in MSSQL
In Microsoft SQL Server (MSSQL), the WITH
clause is commonly used to create Common Table Expressions (CTEs). CTEs are temporary named result sets that simplify complex queries, making them easier to read and write. In this article, we will explore how to use the WITH
clause and how it can be helpful in various scenarios.
Basic Usage of the WITH
Clause
The WITH
clause allows us to temporarily name the result of one or more subqueries. These temporary result sets are called Common Table Expressions (CTEs). The general structure is as follows:
WITH CTE_Name (Column1, Column2, ...)
AS
(
-- Write a SELECT query here
)
SELECT * FROM CTE_Name;
Example:
Suppose we have an Employees
table that lists employee names, salaries, and departments. We want to get a list of employees whose salaries are above the average for their department. We can achieve this with a CTE:
WITH AverageSalaries AS
(
SELECT Department, AVG(Salary) AS AvgSalary
FROM Employees
GROUP BY Department
)
SELECT e.EmployeeName, e.Salary, e.Department
FROM Employees e
JOIN AverageSalaries a ON e.Department = a.Department
WHERE e.Salary > a.AvgSalary;
In this example, we define a CTE called AverageSalaries
, which calculates the average salary for each department. Then, the main query uses this CTE to select employees whose salary is above their department’s average.
Using the WITH
Clause for Recursive CTEs
The WITH
clause can also be used to write recursive queries, which is particularly useful for processing hierarchical data. For example, it can be used to find out how many people report to each employee in a management structure.
Example:
Let’s assume we have an Employees
table where each employee has a manager ID (ManagerID
). We want to calculate how many people report to each employee:
WITH EmployeeHierarchy AS
(
SELECT EmployeeID, ManagerID, 0 AS Level
FROM Employees
WHERE ManagerID IS NULL
UNION ALL
SELECT e.EmployeeID, e.ManagerID, eh.Level + 1
FROM Employees e
JOIN EmployeeHierarchy eh ON e.ManagerID = eh.EmployeeID
)
SELECT * FROM EmployeeHierarchy;
In this example, we define a recursive CTE named EmployeeHierarchy
. The first part (SELECT ... WHERE ManagerID IS NULL
) selects the top-level managers (the highest-level employees). The UNION ALL
part recursively adds the employees under each manager, repeating this process until the hierarchy ends.
Benefits of Using CTEs
- Improves Code Readability: CTEs simplify complex subqueries, making SQL code more readable.
- Reduces Temporary Table Usage: CTEs allow you to name and use results without needing to create and manage temporary tables.
- Suitable for Recursive Queries: CTEs are highly effective when working with recursive data.
- Boosts Performance: In certain scenarios, particularly with complex queries or recursive operations, they can offer performance benefits.
Tips for Using WITH
- You can define multiple CTEs by separating them with commas.
- CTEs are only valid within the scope of the query they are defined in. They do not create a temporary table and are removed from memory once the query is complete.
- Be cautious when using CTEs in performance-sensitive areas, as improper use can lead to performance degradation.
Conclusion
The WITH
clause and CTEs are powerful tools in SQL Server that make data manipulation and querying more efficient and readable. They are highly effective when managing complex subqueries, writing recursive queries, and naming temporary results. By leveraging the advantages of the WITH
clause, you can improve query writing and performance, especially when working with large and complex datasets.