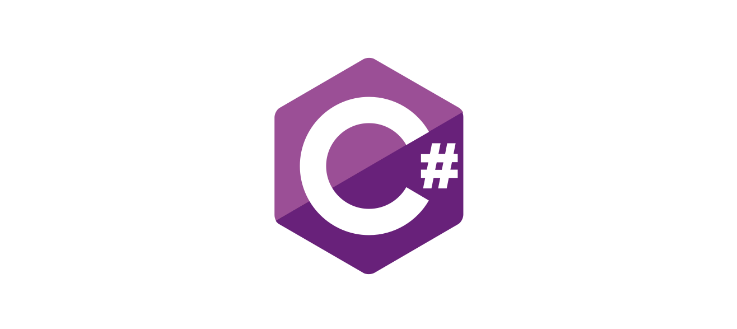
Introduction
C# is a modern programming language based on the object-oriented programming (OOP) paradigm. One of the powerful tools it provides is the “interface” structure. Interfaces can be thought of as a contract that defines how a class should behave and enforces that behavior. In C#, if a class or a struct implements an interface, it must provide implementations for all members defined by that interface. This article will discuss the use of interfaces in C#, their advantages, and some practical examples.
What is an Interface?
An interface is a type that contains only the signatures of members, such as methods, properties, events, or indexers. However, interfaces do not contain any implementation (code blocks); this is the responsibility of the classes that implement them. Interfaces define what a class can do, providing consistency among different classes.
In C#, an interface is defined as follows:
public interface IVehicle
{
void Start();
void Stop();
}
In this example, an interface named IVehicle
is defined, containing two method signatures: Start
and Stop
. Any class that implements this interface must define these methods.
Advantages of Using Interfaces
- Allows Multiple Inheritance: In C#, classes can inherit from only one other class. However, a class can implement multiple interfaces, which provides flexibility similar to multiple inheritance in other languages.
- Loose Coupling: Interfaces promote loose coupling between classes. This means a class does not depend directly on another class but only on an interface. This enhances the testability and reusability of the code.
- Supports Polymorphism: Interfaces support polymorphism, one of the core features of object-oriented programming. An interface allows handling objects of different classes that implement it using the same reference type.
Examples of Using Interfaces
Below are two different classes that implement the IVehicle
interface defined earlier:
public class Car : IVehicle
{
public void Start()
{
Console.WriteLine("Car started.");
}
public void Stop()
{
Console.WriteLine("Car stopped.");
}
}
public class Bicycle : IVehicle
{
public void Start()
{
Console.WriteLine("Bicycle started.");
}
public void Stop()
{
Console.WriteLine("Bicycle stopped.");
}
}
In this example, both the Car
and Bicycle
classes implement the IVehicle
interface. Both classes define the Start
and Stop
methods according to their needs.
Using Interfaces with Polymorphism
Polymorphism is one of the biggest advantages of interfaces. We can create a list of interface types and populate it with objects of different types:
List<IVehicle> vehicles = new List<IVehicle>();
vehicles.Add(new Car());
vehicles.Add(new Bicycle());
foreach (var vehicle in vehicles)
{
vehicle.Start(); // Calls the Start method for both Car and Bicycle
}
In the example above, the vehicles
list is of type IVehicle
and can contain both Car
and Bicycle
objects. In the loop, the Start
method is called for each object, demonstrating the powerful polymorphism provided by interfaces.
Interfaces and Dependency Injection
Interfaces form the foundation of the Dependency Injection (DI) design pattern. DI allows a class to receive its dependencies from the outside, making classes less dependent and more flexible. For example:
public class MotorVehicle
{
private readonly IVehicle _vehicle;
public MotorVehicle(IVehicle vehicle)
{
_vehicle = vehicle;
}
public void RunVehicle()
{
_vehicle.Start();
}
}
In this example, the MotorVehicle
class takes a dependency of any IVehicle
type. This way, the MotorVehicle
class does not depend on specific classes and can work with any type that implements IVehicle
.
Conclusion
Using interfaces in C# is a great way to make your code more flexible, reusable, and maintainable. Interfaces reduce dependencies between objects, enable multiple inheritance, and support polymorphism. When used correctly, interfaces can make your software projects more modular and testable. Particularly in large and complex projects, using interfaces becomes an essential component of software architecture.