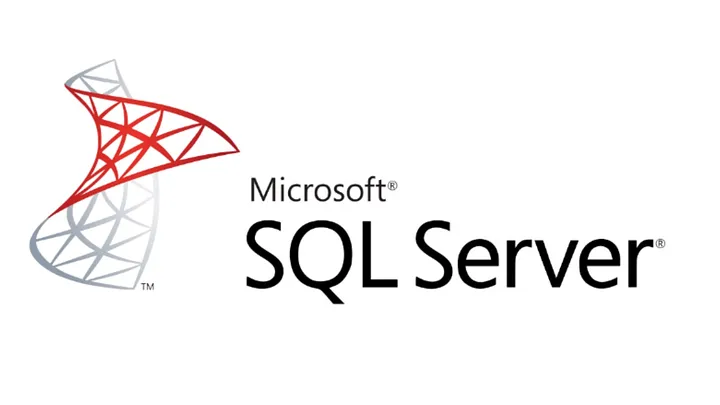
In MSSQL, traditional data manipulation tasks often involve separate INSERT, UPDATE, and DELETE commands. However, when you need to perform these operations in one go on the same dataset, the MERGE
statement—a powerful yet lesser-known feature of MSSQL—comes into play. In this article, we’ll explore how the MERGE
statement can streamline your data updates and make your processes more efficient.
What is MERGE
?
The MERGE
statement is used to combine data from different sources and apply INSERT, UPDATE, or DELETE operations to a target table in one atomic operation. It compares the source data with the target table and determines whether to insert new records, update existing ones, or delete records that no longer exist in the source.
When to Use MERGE
- Data Synchronization: When syncing data between two sources,
MERGE
allows you to insert new records, update existing ones, and delete old records all in one step. - Batch Updates: It’s ideal for situations where you need to update or insert large sets of data into an existing table while cleaning up any obsolete records.
Syntax of the MERGE
Statement
The general structure of a MERGE
statement looks like this:
MERGE INTO TargetTable AS target
USING SourceTable AS source
ON target.KeyColumn = source.KeyColumn
WHEN MATCHED THEN
UPDATE SET target.Column1 = source.Column1
WHEN NOT MATCHED BY TARGET THEN
INSERT (Column1, Column2) VALUES (source.Column1, source.Column2)
WHEN NOT MATCHED BY SOURCE THEN
DELETE;
This structure reflects how MERGE
works:
- It compares rows from the target table with the source data based on a key column.
- If a match is found, it updates the existing rows.
- If no match exists in the target table, it inserts new rows.
- If a row is found in the target table but not in the source, it deletes the target row.
Example: Using MERGE
Let’s say you have a customer database that you need to update with external data. You want to insert new customers, update existing ones, and remove customers no longer in the source data. Here’s how you could do it with MERGE
:
MERGE INTO Customers AS target
USING ExternalCustomerData AS source
ON target.CustomerID = source.CustomerID
WHEN MATCHED THEN
UPDATE SET
target.Name = source.Name,
target.Email = source.Email
WHEN NOT MATCHED BY TARGET THEN
INSERT (CustomerID, Name, Email)
VALUES (source.CustomerID, source.Name, source.Email)
WHEN NOT MATCHED BY SOURCE THEN
DELETE;
This query performs the following actions:
- Updates the
Name
andEmail
of customers already present in theCustomers
table. - Inserts new customers from the source data.
- Deletes customers that are no longer present in the external source.
Benefits of MERGE
- Single-Step Operations: Instead of writing separate INSERT, UPDATE, and DELETE statements,
MERGE
allows you to perform all operations in one go. This simplifies your code and reduces the need for multiple operations. - Data Consistency: Since all operations are handled in a single statement, you ensure that your data is synchronized accurately. This is especially useful in scenarios where multiple operations could lead to inconsistencies if handled separately.
- Reduced Code Redundancy: By using
MERGE
, you avoid the redundancy of writing separate queries for each type of operation. This not only simplifies your code but also improves maintainability.
Performance Considerations
- Efficiency: The
MERGE
statement can improve performance by reducing the number of operations required to update or synchronize data. However, care should be taken with large datasets asMERGE
can be resource-intensive. Proper indexing and query optimization are important for ensuring that your queries run efficiently. - Testing and Monitoring: Although
MERGE
is powerful, it’s important to test its performance with your specific data volumes. Depending on the complexity of your logic and the size of your tables,MERGE
may have varying performance impacts. - Deadlocks: In some cases, especially with large datasets or concurrent operations,
MERGE
can lead to deadlocks. To mitigate this risk, proper transaction handling and indexing strategies should be employed.
Alternatives and Limitations
There are scenarios where using separate INSERT, UPDATE, and DELETE statements might still be preferable. For example, in simpler operations where data volumes are low, breaking down the logic into individual statements could be easier to read and maintain.
Additionally, certain versions of MSSQL have known issues with MERGE
, and its behavior can sometimes be unpredictable depending on the complexity of the query. It’s important to verify compatibility with your SQL Server version and test thoroughly before relying on it for critical operations.
Conclusion
The MERGE
statement in MSSQL is a versatile tool that can simplify complex data synchronization and batch update processes. By allowing you to insert, update, and delete records in one operation, it reduces code complexity and helps maintain data consistency. If you frequently work with large datasets or need to synchronize data from multiple sources, exploring the MERGE
statement could significantly enhance the efficiency of your database operations.
Take the time to test its performance in your specific use case and ensure that it meets your requirements. With careful planning, MERGE
can be a powerful asset in your MSSQL toolkit.