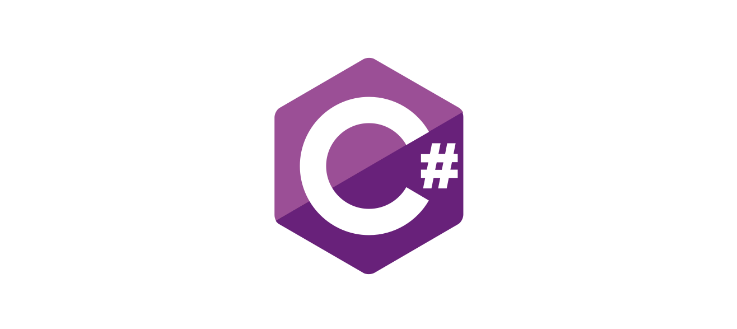
In C#, there are significant differences between async
and normal (synchronous) methods, which can impact performance, user experience, and the overall flow of an application. In this article, we’ll explore the differences between async
and synchronous methods in C#, along with their advantages and disadvantages.
Synchronous (Normal) Methods
Synchronous methods execute tasks sequentially and do not proceed to the next task until the current one is completed. These types of methods are generally suitable for simple operations and improve code readability. For example:
public void DownloadFile()
{
// File download operation
WebClient client = new WebClient();
client.DownloadFile("http://example.com/file.zip", "file.zip");
// After the file is downloaded, other operations
Console.WriteLine("File downloaded.");
}
In the code above, the DownloadFile
method downloads a file and waits for the operation to complete. After the download is finished, the next line is executed.
Advantages
- Simplicity: Synchronous code is easy to write and understand.
- Predictability: Since operations are executed sequentially, debugging is often easier.
Disadvantages
- Blocking: Synchronous methods wait for the operation to complete, blocking the thread they are called on. For example, if a UI thread runs a synchronous method, the user interface may freeze until the operation is completed.
- Inefficiency: Synchronous methods are not suitable for time-consuming operations, as system resources (such as CPU and memory) are used inefficiently during these processes.
Asynchronous (async
) Methods
Asynchronous methods allow execution to continue without waiting for an operation to complete, enabling the application to remain responsive and perform other tasks concurrently. In C#, asynchronous methods are defined with the async
and await
keywords:
public async Task DownloadFileAsync()
{
// File download operation
using (HttpClient client = new HttpClient())
{
await client.GetAsync("http://example.com/file.zip");
}
// After the file is downloaded, other operations
Console.WriteLine("File downloaded.");
}
In the above code, the DownloadFileAsync
method does not block the thread while downloading the file. The waiting is handled by the await
keyword, allowing other operations to continue simultaneously.
Advantages
- Better User Experience: Asynchronous methods allow applications to remain more responsive. The UI operations are not blocked, and user interactions can be handled immediately.
- Improved Performance: During time-consuming I/O operations (e.g., file downloading, database queries), threads are freed up, allowing more efficient use of resources.
- Scalability: On the server side, asynchronous methods provide the ability to handle more requests concurrently.
Disadvantages
- Code Complexity: Asynchronous code can be more complex to write and debug compared to synchronous code.
- Performance Overhead: Asynchronous operations can introduce a slight performance overhead. Using asynchronous code for very simple tasks might be more detrimental than beneficial.
How async
and await
Work
In C#, the async
and await
keywords enable a method to execute asynchronously. The async
keyword indicates that a method can contain asynchronous code, while the await
keyword is used to wait for a specific task to complete. When await
is used, the current thread is freed while the task is running, allowing other operations to be executed.
Example: Asynchronous File Reading
public async Task ReadFileAsync(string filePath)
{
using (StreamReader reader = new StreamReader(filePath))
{
string content = await reader.ReadToEndAsync();
Console.WriteLine(content);
}
}
In this example, the ReadFileAsync
method reads a file asynchronously, preventing the application from freezing during the reading of large files.
When to Use Asynchronous Methods?
Asynchronous methods are particularly useful in the following scenarios:
- Network Operations: Long-running operations like HTTP requests, database transactions, and file downloads.
- File Operations: Reading or writing large files.
- UI Applications: To prevent the user interface from freezing.
However, for CPU-bound tasks (such as intensive computations), synchronous methods might be more appropriate. Asynchronous methods are optimized for I/O-bound operations.
Conclusion
The differences between async
and synchronous methods in C# play a significant role in terms of performance and user experience. Asynchronous methods are a great tool for managing long-running operations and making applications more responsive. However, it’s crucial to use them in the right scenarios and in the right way. Depending on your application’s requirements and use cases, you should carefully evaluate which approach will yield the best results.