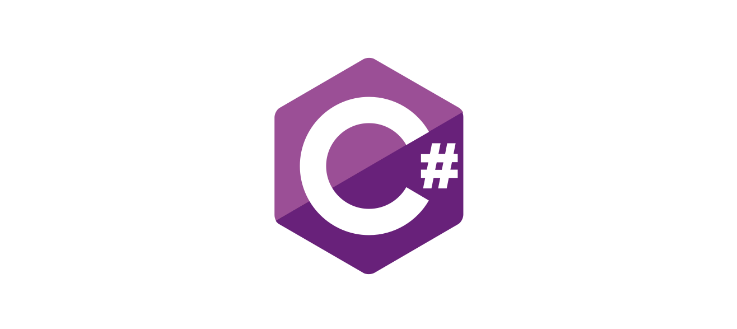
AutoMapper is a powerful library in C# projects that simplifies object mapping operations, making them fast and straightforward. In this article, we will cover a broad range of topics, starting from the basic usage of AutoMapper to in-depth discussions on nested mapping, reverse mapping, conditional mapping, and the usage of profiles.
Table of Contents:
- What is AutoMapper?
- Understanding the fundamental principles of AutoMapper and why it is essential.
- Installing AutoMapper
- Step-by-step guide on adding AutoMapper to your project using NuGet package manager.
- Basic Usage
- Simple mapping operations between two classes with practical usage examples.
var configuration = new MapperConfiguration(cfg => cfg.CreateMap<SourceClass, TargetClass>());
var mapper = new Mapper(configuration);
var sourceObj = new SourceClass { Prop1 = "Value1", Prop2 = "Value2" };
var targetObj = mapper.Map<TargetClass>(sourceObj);
4. Nested Mapping
- Successfully mapping nested objects with clear examples.
var configuration = new MapperConfiguration(cfg =>
{
cfg.CreateMap<SourceClass, TargetClass>();
cfg.CreateMap<SourceNestedClass, TargetNestedClass>();
});
5. Reverse Mapping
- Techniques and examples for mapping objects in both directions.
var configuration = new MapperConfiguration(cfg => cfg.CreateMap<SourceClass, TargetClass>().ReverseMap());
6. Conditional Mapping
- Mapping based on specific conditions with practical code snippets.
var configuration = new MapperConfiguration(cfg =>
{
cfg.CreateMap<SourceClass, TargetClass>()
.ForMember(dest => dest.TargetProp, opt => opt.Condition(src => src.SourceProp != null));
});
7. AutoMapper Profiles
- Organizing mapping operations using profiles and handling more complex scenarios.
public class ProjectProfile : Profile
{
public ProjectProfile()
{
CreateMap<SourceClass, TargetClass>();
// Other mappings...
}
}
8. Performance Improvements
- Configuring AutoMapper for enhanced performance, especially when dealing with large datasets.
var configuration = new MapperConfiguration(cfg =>
{
cfg.AddProfile<ProjectProfile>();
cfg.AllowNullCollections = true;
cfg.ValidateInlineMaps = false;
});
Each topic is accompanied by concise and illustrative code examples, aimed at assisting C# developers in effectively utilizing AutoMapper. Enjoy reading!