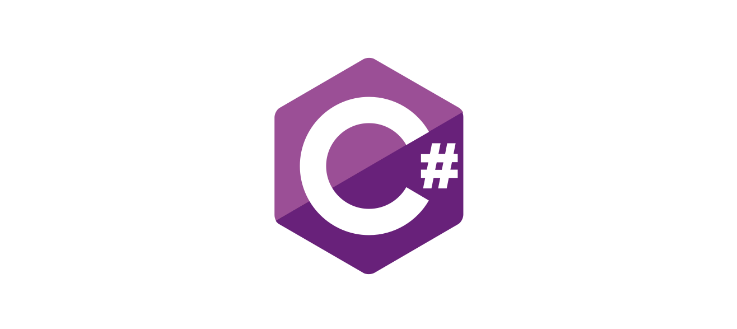
In C#, it’s possible to add new behavior to a class or a struct without modifying the original source. This is achieved using a feature called extension methods. Extension methods allow you to extend existing types (classes, interfaces, or structs) as if they were part of the type itself, without actually modifying the type’s source code.
In this article, we’ll explore how to define and use extension methods, their benefits, and practical use cases where they can make your code cleaner, more modular, and easier to maintain.
What Are Extension Methods?
Extension methods allow you to add new functionality to existing classes or structs, treating them as if the new methods were part of the type. These methods are defined outside the original class, typically in a static class, and can be called as though they were instance methods of the type.
To use extension methods, you need to create a static class and define static methods inside it. The first parameter of these methods should specify the type you’re extending and be prefixed with the this
keyword.
How to Define an Extension Method
Let’s define a simple extension method for the string
type:
public static class StringExtensions
{
public static bool IsNullOrEmpty(this string str)
{
return string.IsNullOrEmpty(str);
}
}
Here, we created a static class StringExtensions
and added a static method IsNullOrEmpty
. The first parameter, this string str
, specifies that this method extends the string
type. Now, you can use this method as if it were a built-in method of the string
class:
string name = null;
bool isEmpty = name.IsNullOrEmpty(); // returns true
Benefits of Extension Methods
- Keep Code Clean and Readable: Extension methods allow you to extend classes or structs without modifying their source. This is particularly useful when you want to refactor code or extend third-party libraries.
- Add New Behavior: When you need to add new behavior to a class but can’t or shouldn’t modify the class itself, extension methods provide a way to do that. For example, you can add new functionality to a library class.
- Reusable Code: Extension methods allow you to create reusable functionality that can be applied to many classes or types. For instance, you can create extension methods for common operations on certain data types and reuse them across different parts of your project.
- Fluent APIs: Extension methods can help create fluent APIs, allowing for method chaining and making your code more expressive and easier to read.
Extension Method Examples
1. Extension Methods for Collections
Let’s say you frequently need to filter collections based on a certain condition. You can define an extension method to handle this:
public static class ListExtensions
{
public static List<T> WhereGreaterThan<T>(this List<T> list, T threshold) where T : IComparable
{
return list.Where(x => x.CompareTo(threshold) > 0).ToList();
}
}
You can use this extension method as follows:
List<int> numbers = new List<int> { 1, 5, 10, 15 };
List<int> greaterThanFive = numbers.WhereGreaterThan(5); // returns 10, 15
2. Fluent API Example
You can use extension methods to support fluent API-style code. For example, here’s a simple string processing scenario:
public static class StringFluentExtensions
{
public static string Append(this string str, string appendString)
{
return str + appendString;
}
public static string ToUpperFluent(this string str)
{
return str.ToUpper();
}
}
These methods can be chained:
string result = "Hello".Append(", World").ToUpperFluent();
// Result: "HELLO, WORLD"
3. Extension Methods for DateTime
When working with the DateTime
class, you might often need to calculate specific days or time intervals. Extension methods can simplify these operations:
public static class DateTimeExtensions
{
public static bool IsWeekend(this DateTime dateTime)
{
return dateTime.DayOfWeek == DayOfWeek.Saturday || dateTime.DayOfWeek == DayOfWeek.Sunday;
}
public static DateTime StartOfDay(this DateTime dateTime)
{
return new DateTime(dateTime.Year, dateTime.Month, dateTime.Day, 0, 0, 0);
}
}
You can now use these methods to make your date-related logic more readable and expressive:
DateTime today = DateTime.Now;
bool isWeekend = today.IsWeekend();
DateTime startOfDay = today.StartOfDay();
Limitations of Extension Methods
- Must Be Defined in Static Classes: Extension methods can only be defined in static classes.
- Cannot Override Methods: Extension methods do not actually modify the class they extend, nor can they override existing methods. If a class has a method with the same name, the class’s own method will take precedence.
- Scope Requirements: You must ensure that the static class containing the extension method is included with a
using
directive wherever you want to use the method.
Conclusion
In C#, extension methods offer a practical and efficient way to extend existing types with new functionality. They enhance the flexibility of the language and make your code more reusable. By leveraging extension methods, you can write more readable, modular, and extensible code. These tools can be especially valuable in large projects or when working with third-party libraries, allowing you to extend functionality without directly modifying the source code.