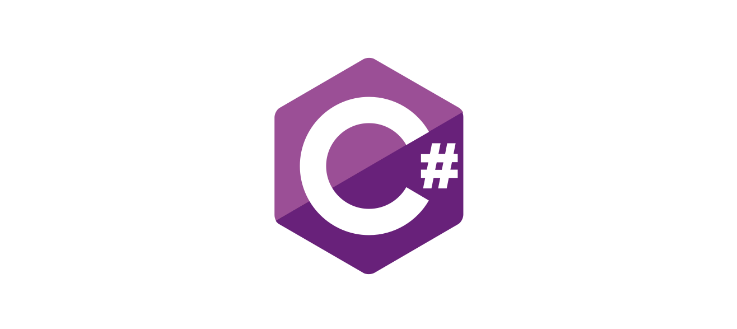
Introduction
In C#, writing performance-oriented code is often a critical goal. When working with large data sets, memory usage and performance become essential considerations. The yield
keyword is a hidden gem in C#, offering developers a way to improve performance, especially when iterating over large collections. In this article, we’ll explore how the yield
keyword allows for lazy iteration, helping developers write cleaner and more efficient code.
What is yield
?
The yield
keyword allows a function to return values one at a time, rather than all at once. Normally, when a method returns a collection, the entire collection is created and returned in memory. However, with yield
, the results are returned incrementally as they are requested. This is known as lazy evaluation, where values are generated only when needed, reducing memory usage and improving performance when handling large data sets.
With yield
, iterators can be created to return data as needed, minimizing memory consumption. This is particularly advantageous when working with large collections or applying filters on them.
How Does yield
Work?
You can use yield return
to return values one at a time in a loop. Since these values are returned incrementally, not all the results are held in memory simultaneously. Here’s a simple example:
public static IEnumerable<int> GetNumbers()
{
for (int i = 1; i <= 5; i++)
{
yield return i;
}
}
This method returns each number in sequence as the loop progresses. Here’s how you would use it:
foreach (var number in GetNumbers())
{
Console.WriteLine(number);
}
The output of this code is:
1
2
3
4
5
In this example, the yield
keyword ensures that GetNumbers()
doesn’t immediately create all numbers. Instead, it generates them one by one as they are needed in the foreach
loop.
Lazy Evaluation: The Power of Deferred Execution
Lazy evaluation with yield
is especially useful when dealing with large data sets. Instead of loading an entire collection into memory, only the required elements are loaded as they are requested. This minimizes memory usage and optimizes processor time.
Example: Processing a Large Data Set Lazily
public static IEnumerable<int> GetLargeDataSet()
{
for (int i = 0; i < 1000000; i++)
{
yield return i;
}
}
In this example, instead of holding millions of elements in memory, elements are returned lazily when the loop iterates over them. This significantly reduces memory consumption when dealing with large data sets.
Filtering with yield
Another useful scenario is filtering data in large collections. With yield return
, you can filter data on the fly without loading the entire collection into memory.
public static IEnumerable<int> GetEvenNumbers()
{
for (int i = 1; i <= 1000; i++)
{
if (i % 2 == 0)
{
yield return i;
}
}
}
This code returns all even numbers between 1 and 1000. However, note that only the even numbers are held in memory as they are found, not the entire range of 1000 numbers.
yield
and IEnumerable
The yield return
keyword is often used with the IEnumerable<T> type. This type allows iteration over collections and yield return
controls how this collection is returned.
You can also use yield break
to terminate the iteration early.
public static IEnumerable<int> GetNumbersWithBreak()
{
for (int i = 1; i <= 10; i++)
{
if (i == 6)
{
yield break; // Stop iteration here
}
yield return i;
}
}
In this example, when the iteration reaches 6, the loop stops and no further numbers are returned.
Benefits of Using yield
- Memory Efficiency: Instead of holding an entire collection in memory, data is generated only when needed.
- Performance Gains: This provides a faster and lighter solution when working with large data sets.
- Cleaner Code: Complex data processing algorithms become easier to understand and maintain.
- Dynamic Data Generation:
yield
provides a flexible way to generate and consume data dynamically.
Drawbacks and Considerations
While yield
provides many benefits, there are some considerations:
- Data is Generated Twice: If you need to iterate over the same collection multiple times,
yield
will regenerate the data on each iteration. For scenarios where precomputed data is needed,yield
may not be the best option. - Performance Cost: For small data sets,
yield
may introduce unnecessary overhead and won’t provide significant performance gains.
Conclusion
The yield
keyword in C# offers great benefits in terms of memory and performance, especially when working with large data sets. By leveraging lazy iteration, you can generate data only when needed, reducing memory usage. In scenarios where memory management and performance are critical, yield
can make a significant difference. It’s a feature worth exploring to make your code both cleaner and more efficient!