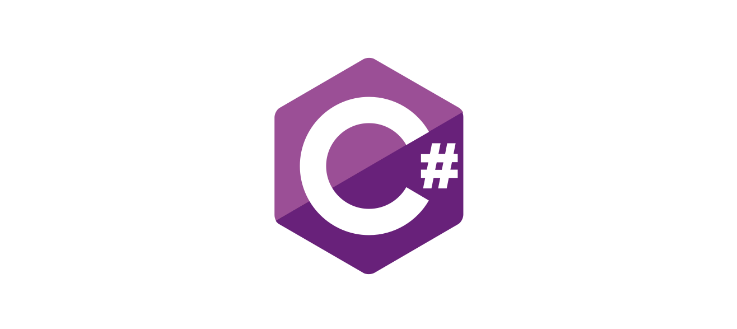
With the evolution of REST APIs, developers have increasingly turned to various tools to better document and present their APIs. One of the most popular tools is Swagger, also known as the OpenAPI Specification (OAS). Swagger provides a standard set of rules for documenting APIs, defining endpoints, data models, sample requests and responses, and error states. This documentation is invaluable for developers, partners, and customer developers alike. In this article, we’ll dive deep into customizing Swagger for .NET 7 and beyond to enhance your API documentation experience.
What is Swagger and OpenAPI?
Swagger (OpenAPI) is a widely accepted standard for documenting REST APIs. It provides a clear and concise way to define API endpoints, request/response formats, data models, and error handling. This documentation is crucial for developers, as it facilitates both development and integration processes.
Swagger Support in .NET 7
.NET 7 brings several new features and enhancements that make it even easier to customize and improve API documentation using Swagger. In .NET 7 projects, the most common library for integrating Swagger is Swashbuckle.AspNetCore. This library provides a seamless integration of Swagger with ASP.NET Core.
Setting Up Swagger
The first step is to add the Swashbuckle package to your project:
dotnet add package Swashbuckle.AspNetCore
Next, you need to configure the Swagger service and middleware in your Program.cs
file:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.MapControllers();
app.Run();
With this basic setup, you can run your application and access the Swagger UI interface to document your APIs. However, for most projects, this basic setup is not enough. Let’s explore ways to customize Swagger to better fit your needs.
Customizing Swagger
1. Customizing API Information
You can customize the title, description, and version information of your Swagger document by modifying the SwaggerGen
configuration:
builder.Services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo
{
Version = "v1",
Title = "Sample API",
Description = "This API is built with .NET 7.",
TermsOfService = new Uri("https://example.com/terms"),
Contact = new OpenApiContact
{
Name = "API Developer",
Email = "developer@example.com",
Url = new Uri("https://example.com/contact"),
},
License = new OpenApiLicense
{
Name = "MIT License",
Url = new Uri("https://example.com/license"),
}
});
});
This configuration provides more information about your API in the Swagger UI.
2. Adding Authentication and Security Definitions
You can customize Swagger UI to include user authentication. For example, if you use JWT tokens for authentication, you can add the following configuration:
builder.Services.AddSwaggerGen(c =>
{
c.AddSecurityDefinition("Bearer", new OpenApiSecurityScheme
{
In = ParameterLocation.Header,
Description = "Please enter 'Bearer' followed by your token.",
Name = "Authorization",
Type = SecuritySchemeType.ApiKey,
Scheme = "Bearer"
});
c.AddSecurityRequirement(new OpenApiSecurityRequirement()
{
{
new OpenApiSecurityScheme
{
Reference = new OpenApiReference
{
Type = ReferenceType.SecurityScheme,
Id = "Bearer"
},
Scheme = "oauth2",
Name = "Bearer",
In = ParameterLocation.Header,
},
new List<string>()
}
});
});
This addition allows the Swagger UI to provide an “Authorize” button for user authentication.
3. Grouping and Filtering Endpoints
Swagger allows you to group endpoints to make them more manageable, especially in large projects. You can use the c.TagActionsBy
method to group by controller name or route:
builder.Services.AddSwaggerGen(c =>
{
c.TagActionsBy(api => api.GroupName ?? api.HttpMethod);
});
This categorizes your endpoints more intuitively in the Swagger UI.
4. Customizing Swagger UI
You can also customize the Swagger UI to suit your needs better. Instead of working with the default theme and layout, you can modify the index.html
file or inject your CSS and JavaScript files:
app.UseSwaggerUI(c =>
{
c.InjectStylesheet("/swagger-ui/custom.css");
c.InjectJavascript("/swagger-ui/custom.js");
c.DocumentTitle = "Customized Swagger UI";
});
5. Advanced Documentation with XML Comments
XML comments can make your Swagger documentation more informative. To enable XML comments in your project and include them in the Swagger configuration, follow these steps:
- Enable XML Comments:Add the following lines to your
csproj
file:
<PropertyGroup>
<GenerateDocumentationFile>true</GenerateDocumentationFile>
</PropertyGroup>
2. Include the Comment File in Swagger:
Add the following in your Program.cs
:
var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml";
var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile);
c.IncludeXmlComments(xmlPath);
With this setup, the Swagger UI will display more detailed information about methods and parameters.
Conclusion
Swagger is a powerful tool for API documentation, and when used with .NET 7, it significantly enhances the development process. In this article, we covered a range of customization options, from basic setup to advanced configurations. By improving your API documentation with Swagger, you can enhance the developer experience and make your workflows more efficient.
For further information on other customizations you can achieve with Swagger, I recommend checking out the official Swagger documentation.